Contributed by Renan de Lima in #44405.
Routing in Symfony applications is usually simple and consists of mapping some controller method to some URL path. However, sometimes you need to evaluate complex conditions to decide if some incoming URL should match a given controller. That's why Symfony allows using expressions to match routes. In Symfony 6.1 we've improved routing conditions so you can also call services inside those expressions. To do that, use the new service() function and pass the name of the service to call:
// src/Controller/DefaultController.php
namespace App\Controller;
use Symfony\Bundle\FrameworkBundle\Controller\AbstractController; use Symfony\Component\HttpFoundation\Response; use Symfony\Component\Routing\Annotation\Route;
class DefaultController extends AbstractController {
[Route(
'/some-path',
name: 'some_name',
condition: "service('some_service').someMethod()",
)]
public function someControllerMethod(): Response
{
// ...
}
}
By default, and for performance reasons, you cannot call any of the services defined in your application. Instead, you must add the routing.condition_service tag or the #[AsRoutingConditionService] attribute to those services that will be available in route conditions:
use Symfony\Bundle\FrameworkBundle\Routing\Attribute\AsRoutingConditionService;
// ...
[AsRoutingConditionService(alias: 'some_service')]
class SomeService { public function someMethod(): bool { // ... } }
The alias option defines how this service will be referred to inside the expression, so you don't have to use the full service name (which is usually too long).
Sponsor the Symfony project.
Zaloguj się, aby dodać komentarz
Inne posty w tej grupie
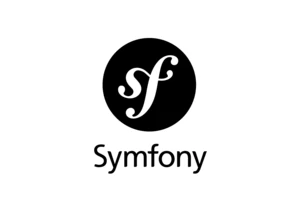
Symfony's bridge packages integrate third-party services, such as mailers, notifiers, and translation providers, into Symfony applications. With more than 120 bridges available today, Symfony supports
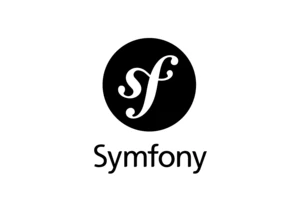
Symfony Messenger component keeps evolving to meet the needs of complex, modern applications. In Symfony 7.3, we're introducing several powerful features to it.
Run Process Using the Shell… https://s
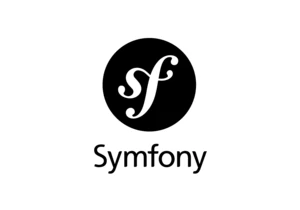
The Routing component provides an impressive list of features to map incoming URLs to your application code. Symfony 7.3 pushes it even further with a set of new features that improve developer experi
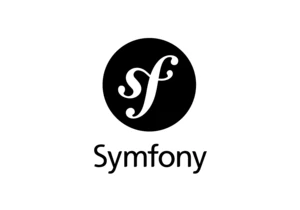
Contributed by Mathias Arlaud in
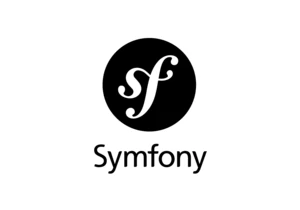
Affected versions
Symfony UX symfony/ux-live-component and symfony/ux-twig-component versions <2.25.1 are affected by this security issue.
The issue has been fixed in the 2.25.1 version of these
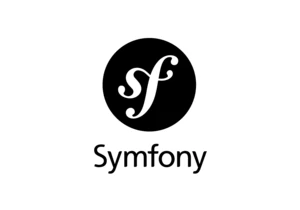
Symfony has been reducing the need for configuration in applications for several years now. Thanks to PHP attributes, you can now configure most things alongside the relevant code, removing the need f
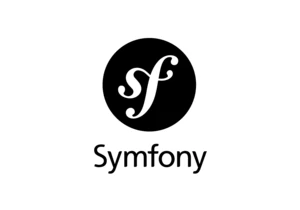
This week, development activity focused on polishing Symfony 7.3 ahead of its final release in two weeks. We also continued publishing articles highlighting the new features of Symfony 7.3 and shared