PHP Enumerations, or "PHP enums" for short, is one of the most anticipated new features of PHP 8.1 (released on November 25, 2021). Their most common use case is to group several related values. In previous PHP versions you had to define a series of related constants to achieve that. In PHP 8.1 you can do this:
1
2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 // Defining the enumeration and its values namespace App\Config;
enum TextAlign { case Left; case Center; case Right; }
// Using the enumeration as a data type use App\Config\TextAlign;
class SomeClass { public function setTextAlignment(TextAlign $alignment) { // ... } }
// Using the enum in action $someObject->setTextAlignment(TextAlign::Center);
PHP Enumerations are modeled as ADT (Algebraic Data Type) values, so they can define methods, implement interfaces, define values, etc. In Symfony 5.4 we've added support for PHP enums in different components.
PHP Enums Support in Symfony Forms
Contributed by Alexander M. Turek
in #43095.
The Form component has added a new EnumType field so you can represent PHP enums as a selection of values. Consider this example:
1
2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 enum Suit: string { case Hearts = 'H'; case Diamonds = 'D'; case Clubs = 'C'; case Spades = 'S'; }
enum Rank: string { case Ace = 'A'; case King = 'K'; case Queen = 'Q'; case Jack = 'J'; case Ten = 'X'; case Nine = '9'; case Eight = '8'; case Seven = '7'; case Six = '6'; case Five = '5'; case Four = '4'; case Three = '3'; case Two = '2'; }
final class Card { public ?Suit $suit = null; public ?Rank $rank = null; }
Use the new EnumType class to represent these PHP enums as form fields:
1
2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 // src/Form/Type/CardType.php namespace App\Form\Type;
use App\Config\Suit; use App\Config\Rank; use Symfony\Component\Form\AbstractType; use Symfony\Component\Form\Extension\Core\Type\EnumType; use Symfony\Component\Form\Extension\Core\Type\SubmitType; use Symfony\Component\Form\FormBuilderInterface;
class CardType extends AbstractType { public function buildForm(FormBuilderInterface $builder, array $options): void { $builder ->add('suit', EnumType::class, ['class' => Suit::class, 'expanded' => true]) ->add('rank', EnumType::class, ['class' => Rank::class]) ->add('submit', SubmitType::class) ; }
// ...
}
This is how the form will look like in Symfony 5.4:
PHP Enums Support in Symfony Serializer
Contributed by Alexandre Daubois
in #40830.
PHP enums that define scalar values for their entries are called "backed enums". When properties use that kind of PHP enum, the Symfony Serializer component can normalize and denormalize those properties:
1
2 3 4 5 6 7 8 9 10 11 final class Card { public Suit $suit; }
$card = new Card(); $card->suit = Suit::Diamonds; $serialized = $this->serializer->serialize($card, 'json') // expected: '{ "suit": "D" }'
$this->serializer->deserialize($serialized, Card::class); // expected: Card->suit === Suit::Diamonds
PHP Enums Support in other components Alexandre Daubois also contributed other Pull Requests to add PHP enums support in other Symfony components: Pull Request #40857 added enums support when dumping the Dependency Injection container; Pull Request #41072 added enums support to VarExporter component. PHP Enumerations are fantastic and Symfony 5.4 will fully support them since day one.
Sponsor the Symfony project.
Login to add comment
Other posts in this group
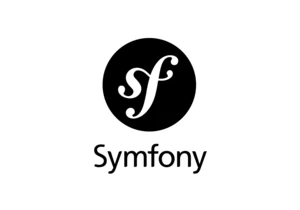
SymfonyOnline June 2025 is almost here, starting in almost 2 months on:
June 10-11: Workshop days. It is possible to attend 1 two-day training or 2 one-day trainings. June 12-13: Online confe

Contributed by Nate Wiebe in

SymfonyOnline June 2025 is almost here, starting in almost 2 months on:
June 10-11: Workshop days. It is possible to attend 1 two-day training or 2 one-day trainings. June 12-13: Online confe
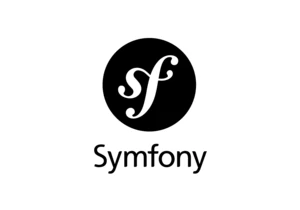
The Validator component provides dozens of constraints ready to use in your applications. In Symfony 7.3, we've added two new constraints to the list.
Slug Constraint
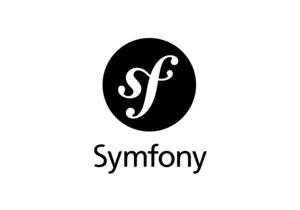
SymfonyOnline June 2025 is almost here, starting in almost 2 months on:
June 10-11: Workshop days. It is possible to attend 1 two-day training or 2 one-day trainings. June 12-13: Online confe

Contributed by Jérôme Tamarelle in
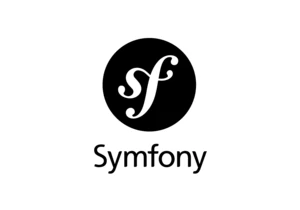
This week, we kicked off the New in Symfony 7.3 blog series, highlighting all the exciting new features coming in this release. We also unveiled more details about some of the SymfonyOnline June 2025